c testing static sealed class|how to test a static function : service There are 2 ways to do this. Include the c source file into the unit testing source file, so the static method now is in the scope of unit testing source file and callable. Do a trick: . Resultado da Vestido Decote Costas com as melhores condições você encontra no site do Magalu. Confira!
{plog:ftitle_list}
Resultado da 2M Followers, 0 Following, 731 Posts - See Instagram photos and videos from Filip Madalina Ioana (@mady_gio)
Moles may be used to detour any .NET method, including non-virtual/static methods in sealed types. UPDATE: there is a new framework called "Fakes" in the upcoming VS 11 release that is designed to replace Moles: There are 2 ways to do this. Include the c source file into the unit testing source file, so the static method now is in the scope of unit testing source file and callable. Do a trick: .
In Objective-C, static methods, a.k.a class methods, can be mocked using OCMock. Here is an example of code that calls a static method on the Helper class: By seamlessly integrating mocking techniques into your unit testing workflow, you can effectively test code that relies on static classes in C#. Achieve better test coverage, . Also allows for testing if a static Func was called from another method since static methods can not be mocked. Easy to refactor existing methods to Funcs/Actions since the syntax for calling a method on the call site .
As soon as you have a need to "mock" a sealed class, you should at least review your design. However, in your case, I would consider refactoring your code and removing direct . Sealed classes or static methods can't be stubbed using stub types because stub types rely on virtual method dispatch. For such cases, use shim types as described in Use . Learn when static methods can’t be unit tested and how to use wrapper classes and the Moq and xUnit frameworks to unit test them when they can
What is a Sealed Class? Sealed classes in C# restrict inheritance. When we define our class as a sealed class, we cannot inherit from it anymore. We can use the sealed . Inner Class In Java, one can define a new class inside any other class. Such classes are known as Inner class. It is a non-static class, hence, it cannot define any static members in itself. Every instance has access to .Output: Explanation: The above program has two classes: the MathHelper class, which is static, and the Main class.The MathHelper class is static, meaning we cannot create objects for that class. This class contains only static methods. .
If it is an internal class then it must not be getting used in isolation. Therefore you shouldn't really be testing it apart from testing some other class that makes use of that object internally. Just as you shouldn't test private members of a class, you shouldn't be testing internal classes of a DLL.
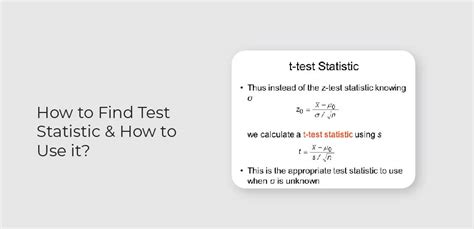
how to test static in c
It can take methods in sealed classes and replace virtual calls with direct calls - and it can also do this for non-sealed classes if it can figure out it's safe to do so. In such a case (a sealed class that the CLR couldn't otherwise detect as safe to devirtualise), a sealed class should actually offer some kind of performance benefit. In this article. When applied to a class, the sealed modifier prevents other classes from inheriting from it. In the following example, class B inherits from class A, but no class can inherit from class B.. class A {} sealed class B : A {} You can also use the sealed modifier on a method or property that overrides a virtual method or property in a base class. Inheritance in multiplatform projects. There is one more inheritance restriction in multiplatform projects: direct subclasses of sealed classes must reside in the same source set.It applies to sealed classes without the expected and actual modifiers.. If a sealed class is declared as expect in a common source set and have actual implementations in platform . To test your internal code this behaviour is exactly what you want. If this is too much, you can add this attribute in a specific class and only allow access to the internal methods of this class. As soon as you recompile your assembly, the code in your test assembly can access your internal methods: .Net Core
Unit tests should test the interface of the method or class, not the implementation. So it should not matter if the method uses a sealed class, it can just be tested as part of the method. While your test might test more than a single method, that is fine in my opinion.In this tutorial, we will learn about the sealed class and method in C# with the help of examples. 36% off. Learn to code solving problems and . The Pens class of the System.Drawing namespace is one of the examples of the sealed class. The Pens class has static members that represent the pens with standard colors. Pens.Blue represents a pen .
public sealed class Husky : Animal { public override void DoNothing() { } public override int GetAge() => 11; } Again, we inherit from the Animal class and override the two methods. It is important to note that the Husky class is sealed since it is a specific dog breed. On the other hand, the Bear class is open for inheritance because we could have multiple types .
Sealed Class and Sealed Methods in C# with Examples. In this article, I am going to discuss Sealed Class and Sealed Methods in C# with Examples.Please read our previous article where we discussed Partial Classes and Partial Methods in C#.At the end of this article, you will understand what exactly Sealed Class and Sealed Methods in C# are and when and how to . In C++, a "static class" has no meaning. The nearest thing is a class with only static methods and members. Using static methods will only limit you. What you want is, expressed in C++ semantics, to put your function (for it is a function) in a namespace. Edit 2011-11-11. There is no "static class" in C++. Sealed classes are used to restrict the users from inheriting the class. A class can be sealed by using the sealed keyword. The keyword tells the compiler that the class is sealed, and therefore, cannot be extended. No class can be derived from a sealed class. The following is the syntax of a sealed class : sealed class class_name { // data members And then today, we had an in-house static class that needed testing. I did the same thing, but with a nastygram: // This exists solely for testing, but suggests [the static class] should not be static // if we want to test its logic. That is, the second one is likely a code smell, and demands a refactor if we're serious about testing.
Allows classes to be sealed and for the elimination of virtual methods that are required by popular mocking frameworks like Moq. Sealing classes and eliminating virtual methods makes them candidates for inlining . For your specific question, options A and B are effectively equal, i.e. you superimpose an interface on a class which has none, by wrapping it in a class that does have the interface. It's less ideal than just putting the . Abstract yourself from third-party libraries by adding an adapter class that's so simple, you could test it by inspection. Rather than extending your target class, just compose your new class over the top of it. . In order to mock sealed classes, non-virtual methods or static member is generally required a mocking framework that is profiler . Based on what you've described, I wouldn't bother with mocking; I'd just use an interface, and use a NOOP. The static class can be just that - a static class, but you can come up with an interface for the log function, and an implementation that .
Ensuring Singleton Integrity: By declaring the class sealed, we ensure the integrity of the Singleton instance. If the class was not sealed, a derived class could add new static members, introduce a new access point to the Singleton instance, or even create new instances independently of the original Singleton control mechanism. No, you can't extend a sealed class in any legitimate way. TypeMock allows you to mock sealed classes, but I doubt that they'd encourage you to use the same technique for production code. If a type has been sealed, that means .
Now the actual scenario :- a sealed class having 3 to 4 nested class and there exist a inheritance relationship between them and now i want to use the member function of nested class present in the sealed class outside the namespace . i can do so , but the question here is how to instantiate a class that has private constructor and without . Static classes are sealed and therefore can't be inherited. They can't inherit from any class or interface except Object. Static classes can't contain an instance constructor. However, they can contain a static constructor. Non-static classes should also define a static constructor if the class contains static members that require non-trivial .Decorator requires you to wrap any calls to members on the wrapped class. Extensions present themselves as just extra members on the original class themselves. So if I have a class with 30 public methods if I wanted to make a wrapper class I would have to create up to 30 public methods to call into the class, plus whatever additional methods I . Note that static methods cannot be mocked easily. As an example, if you have two classes named A and B and class A uses a static member of class B, you wouldn’t be able to unit test class A in .
Prevent Inheritance: Restrict other classes from inheriting to maintain design integrity and avoid issues with subclassing. Security and Stability: Protect critical functionality, ensuring consistent and secure implementation. Performance Optimization: Allow .NET runtime optimizations due to no further derivations. Clear Class Hierarchy: Maintain a well-defined class structure for better . Sub2 allows any number of subclasses, so it seems like it releases control of the types you can create. However, you strictly limit the immediate subclasses of the sealed class Super.That is, Super still allows only the direct subclasses Sub1 and Sub2. A JDK 16 record (described in a previous article in this series) can also be used as a sealed implementation of .
Clarification: I am talking about the scenario when you want to unit test a method and that method calls a static method in a different unit/class. By most definitions of unit testing, if you just let the method under test call the static method in the other unit/class then you are not unit testing, you are integration testing. (Useful, but not .
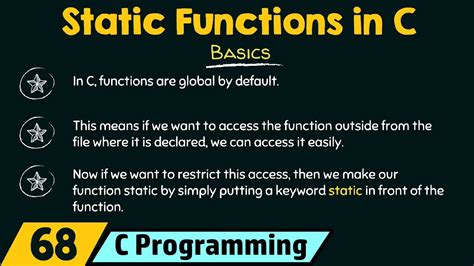
how to test a static function
webVer Divertida-Mente 2 (Inside Out 2) | Disney+. Preparem-se para uma inundação de novas emoções.
c testing static sealed class|how to test a static function